Introduction to the DS1307 Real-Time Clock
The DS1307 is a popular and widely used Real-Time Clock (RTC) integrated circuit (IC) that provides precise timekeeping functionality to electronic projects. This low-power chip, developed by Maxim Integrated (formerly Dallas Semiconductor), is designed to maintain accurate time and date information even when the main power supply is interrupted. The DS1307 is commonly used in a variety of applications, including embedded systems, data logging, automation, and any project that requires reliable timekeeping.
In this comprehensive guide, we will explore the DS1307 pinout, its features, and how to integrate it into your projects. We will also cover the basics of interfacing the DS1307 with microcontrollers, such as Arduino, and provide code examples to help you get started.
DS1307 Features and Specifications
Before diving into the pinout details, let’s take a look at the key features and specifications of the DS1307 RTC:
- Accurate timekeeping with seconds, minutes, hours, day, date, month, and year
- 56-byte non-volatile RAM (NVRAM) for data storage
- I2C serial interface for easy communication with microcontrollers
- Low power consumption: less than 500nA in battery-backup mode
- Wide operating voltage range: 4.5V to 5.5V
- Operating temperature range: -40°C to +85°C
- Automatic leap year compensation valid up to 2100
- Selectable square wave output signal
DS1307 Pinout and Pin Description
The DS1307 is available in an 8-pin DIP (Dual Inline Package) or an 8-pin SOIC (Small Outline Integrated Circuit) package. The pinout for both packages is identical, making it easy to use the DS1307 in various projects. Let’s take a closer look at each pin and its function:
Pin Number | Pin Name | Description |
---|---|---|
1 | X1 | 32.768 kHz crystal connection |
2 | X2 | 32.768 kHz crystal connection |
3 | VBAT | Battery input for backup power supply |
4 | GND | Ground |
5 | SDA | Serial Data (I2C bus) |
6 | SCL | Serial Clock (I2C bus) |
7 | SQW/OUT | Square Wave/Output Driver |
8 | VCC | Power supply (4.5V to 5.5V) |
X1 and X2 (Pins 1 and 2)
The X1 and X2 pins are used to connect a 32.768 kHz crystal to the DS1307. This crystal provides the reference clock for the RTC to maintain accurate timekeeping. The crystal should be a low-cost, standard 32.768 kHz quartz crystal with a load capacitance of 12.5 pF.
VBAT (Pin 3)
The VBAT pin is used to connect a backup power supply, typically a 3V lithium coin cell battery (such as CR2032), to maintain timekeeping when the main power supply is absent. When the main power is disconnected, the DS1307 automatically switches to the backup power source to continue timekeeping.
GND (Pin 4)
The GND pin is the ground connection for the DS1307. It should be connected to the common ground of your system.
SDA and SCL (Pins 5 and 6)
The SDA (Serial Data) and SCL (Serial Clock) pins are used for I2C communication between the DS1307 and a microcontroller. The I2C bus is a simple, two-wire interface that allows multiple devices to communicate using only two lines: SDA for data transfer and SCL for clock synchronization. The DS1307 operates as a slave device on the I2C bus, with a fixed address of 0x68.
SQW/OUT (Pin 7)
The SQW/OUT pin can be configured to output a square wave signal with one of four selectable frequencies: 1 Hz, 4 kHz, 8 kHz, or 32 kHz. This feature is useful for generating periodic interrupts or as a reference clock for other parts of your system. If the square wave output is not needed, this pin can be left unconnected.
VCC (Pin 8)
The VCC pin is the main power supply input for the DS1307. It should be connected to a stable power source within the range of 4.5V to 5.5V, such as the 5V pin on an Arduino board.
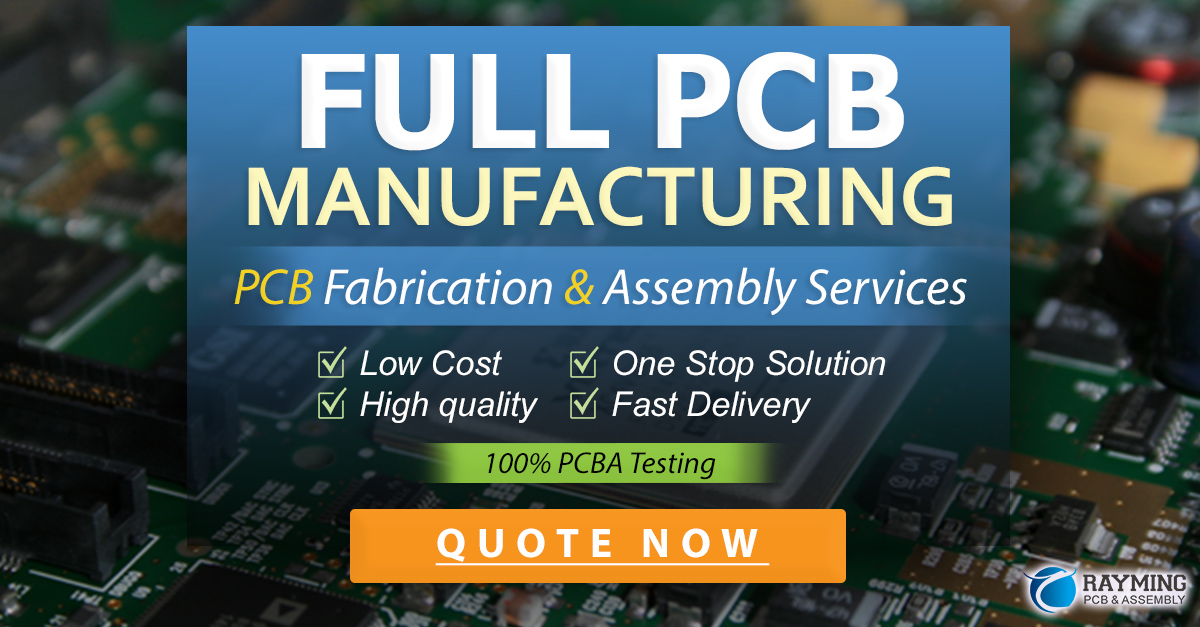
Interfacing the DS1307 with Arduino
Now that we have a clear understanding of the DS1307 pinout and its functions, let’s explore how to interface the RTC with an Arduino board. We will use the I2C communication protocol to read and write data to the DS1307.
Hardware Connections
To connect the DS1307 to an Arduino, you will need to make the following connections:
DS1307 Pin | Arduino Pin |
---|---|
VCC | 5V |
GND | GND |
SDA | A4 (SDA) |
SCL | A5 (SCL) |
If you are using a different Arduino board or a different I2C port, make sure to check the board’s pinout diagram and use the appropriate SDA and SCL pins.
Installing the RTClib Library
To simplify the process of communicating with the DS1307, we will use the RTClib library, which provides an easy-to-use interface for reading and writing data to the RTC. Follow these steps to install the library:
- Open the Arduino IDE
- Go to Sketch -> Include Library -> Manage Libraries
- Search for “RTClib” in the search bar
- Click on the “RTClib by Adafruit” library and click “Install”
Example Code: Setting and Reading the Time
Here’s an example Arduino sketch that demonstrates how to set the current time on the DS1307 and read it back:
#include <Wire.h>
#include <RTClib.h>
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
Wire.begin();
rtc.begin();
// Set the current time (uncomment the line below and modify as needed)
// rtc.adjust(DateTime(2023, 4, 15, 10, 30, 0));
}
void loop() {
DateTime now = rtc.now();
Serial.print("Date: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" Time: ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
delay(1000);
}
In this example, we first include the necessary libraries: Wire.h for I2C communication and RTClib.h for the DS1307 RTC functions. We create an instance of the RTC_DS1307 class called rtc
.
In the setup()
function, we initialize the Serial communication, the I2C bus (Wire), and the RTC. If you need to set the current time, uncomment the rtc.adjust()
line and modify the date and time parameters according to your needs.
In the loop()
function, we read the current date and time from the DS1307 using the rtc.now()
function, which returns a DateTime object. We then print the date and time components to the Serial monitor.
Example Code: Using the Square Wave Output
If you want to use the square wave output feature of the DS1307, you can enable it using the rtc.squareWave()
function. Here’s an example sketch that enables the square wave output at 1 Hz:
#include <Wire.h>
#include <RTClib.h>
RTC_DS1307 rtc;
void setup() {
Wire.begin();
rtc.begin();
// Set the square wave output to 1 Hz
rtc.squareWave(DS1307_1HZ);
}
void loop() {
// Your code here
}
In this example, we enable the square wave output at 1 Hz using the rtc.squareWave(DS1307_1HZ)
function. You can choose from four different frequencies: DS1307_1HZ, DS1307_4KHZ, DS1307_8KHZ, and DS1307_32KHZ.
DS1307 and Non-Volatile RAM (NVRAM)
In addition to its timekeeping capabilities, the DS1307 also includes 56 bytes of non-volatile RAM (NVRAM). This memory can be used to store small amounts of data that persist even when power is removed from the RTC.
Reading and Writing to NVRAM
To read and write data to the DS1307’s NVRAM, you can use the rtc.readnvram()
and rtc.writenvram()
functions provided by the RTClib library. Here’s an example sketch that demonstrates how to write and read data to/from the NVRAM:
#include <Wire.h>
#include <RTClib.h>
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
Wire.begin();
rtc.begin();
// Write data to NVRAM
uint8_t data[] = {0x12, 0x34, 0x56, 0x78};
rtc.writenvram(0, data, sizeof(data));
// Read data from NVRAM
uint8_t readData[4];
rtc.readnvram(0, readData, sizeof(readData));
// Print the read data
for (int i = 0; i < sizeof(readData); i++) {
Serial.print("0x");
if (readData[i] < 0x10) {
Serial.print('0');
}
Serial.print(readData[i], HEX);
Serial.print(' ');
}
Serial.println();
}
void loop() {
// Your code here
}
In this example, we write an array of four bytes (data
) to the NVRAM starting at address 0 using the rtc.writenvram()
function. We then read the same four bytes from the NVRAM using the rtc.readnvram()
function and store them in the readData
array. Finally, we print the read data to the Serial monitor in hexadecimal format.
Troubleshooting Common Issues
Incorrect Time and Date
If you notice that the time and date reported by the DS1307 are incorrect, there are a few things you can check:
- Make sure that the battery is properly connected to the VBAT pin and has sufficient charge.
- Check that the crystal is properly connected to the X1 and X2 pins and that it is a 32.768 kHz crystal with the correct load capacitance.
- Verify that you have set the correct time and date using the
rtc.adjust()
function.
I2C Communication Problems
If you encounter issues with I2C communication between the Arduino and the DS1307, consider the following:
- Double-check the wiring connections between the Arduino and the DS1307, ensuring that the SDA and SCL pins are connected correctly.
- Make sure that the I2C bus is properly initialized in your Arduino sketch using the
Wire.begin()
function. - Check if there are any other devices on the I2C bus that might be causing conflicts. If necessary, try disconnecting other devices to isolate the issue.
Compilation Errors
If you encounter compilation errors related to the RTClib library, ensure that you have installed the library correctly and that you are using the most up-to-date version. If the issue persists, try reinstalling the library or checking the library’s documentation for any specific requirements or known issues.
Conclusion
The DS1307 Real-Time Clock is a versatile and reliable solution for adding timekeeping functionality to your electronic projects. By understanding the DS1307 pinout, features, and how to interface it with microcontrollers like Arduino, you can easily integrate accurate timekeeping into your designs.
In this guide, we covered the basics of the DS1307, including its pinout, features, and specifications. We also explored how to connect the DS1307 to an Arduino and provided example code for setting and reading the time, as well as using the square wave output and NVRAM features.
With this knowledge, you can now confidently use the DS1307 in your projects, whether you are building a data logger, an automated system, or any other application that requires reliable timekeeping.
FAQ
- Q: Can I use the DS1307 with a 3.3V microcontroller?
A: Yes, the DS1307 is designed to work with a wide range of voltages, including 3.3V. However, you should ensure that the I2C bus voltage levels are compatible with your microcontroller. You may need to use a level shifter if your microcontroller’s I2C pins are not 5V tolerant. - Q: How accurate is the DS1307 RTC?
A: The accuracy of the DS1307 depends on the quality of the 32.768 kHz crystal and the operating environment. Typically, the DS1307 has an accuracy of ±2ppm at 25°C, which translates to an error of about ±1 minute per year. However, factors such as temperature variations and crystal aging can affect the accuracy over time. - Q: Is the DS1307 still available for purchase?
A: Yes, the DS1307 is still widely available from various electronic component suppliers. However, it’s worth noting that there are newer RTC chips available, such as the DS3231, which offer improved accuracy and additional features. - Q: Can I use the DS1307 without a backup battery?
A: Yes, you can use the DS1307 without a backup battery, but it will not maintain the time and date when the main power supply is disconnected. If timekeeping is critical for your application, it is recommended to use a backup battery to ensure uninterrupted operation. - Q: How do I set the initial time on the DS1307?
A: To set the initial time on the DS1307, you need to use thertc.adjust()
function provided by the RTClib library. You can pass the desired date and time as parameters to this function, as shown in the example code in this guide. Make sure to uncomment the relevant line and modify the date and time according to your needs.