In the world of software development, creating a new component is a fundamental task that developers encounter regularly. Whether you’re working on a web application, a mobile app, or a desktop software, components are the building blocks that make up the user interface and functionality of your application. In this article, we’ll explore the process of creating a new component, from understanding what a component is to implementing it in your project. We’ll also discuss best practices, common pitfalls, and tips for ensuring that your component is reusable, maintainable, and efficient.
Table of Contents
- Introduction to Components
- What is a Component?
- Types of Components
- Importance of Components in Software Development
- Planning Your Component
- Identifying the Need for a New Component
- Defining the Component’s Purpose
- Gathering Requirements
- Sketching the Component’s Design
- Designing the Component
- Choosing the Right Technology Stack
- Designing the Component’s Architecture
- Creating a Mockup or Prototype
- Considering User Experience (UX)
- Implementing the Component
- Setting Up the Development Environment
- Writing the Component’s Code
- Testing the Component
- Integrating the Component into Your Application
- Best Practices for Component Development
- Writing Clean and Maintainable Code
- Ensuring Reusability
- Optimizing Performance
- Documenting Your Component
- Common Pitfalls and How to Avoid Them
- Overcomplicating the Component
- Ignoring Cross-Browser Compatibility
- Neglecting Accessibility
- Failing to Test Thoroughly
- Conclusion
- Recap of Key Points
- Final Thoughts on Creating a New Component
1. Introduction to Components
What is a Component?
A component is a self-contained, reusable piece of code that encapsulates a specific functionality or user interface element. Components can range from simple buttons and input fields to complex data tables and navigation menus. They are designed to be modular, meaning they can be easily integrated into different parts of an application without affecting the overall structure.
Types of Components
Components can be categorized based on their functionality and complexity:
- UI Components: These are visual elements that users interact with, such as buttons, forms, modals, and cards.
- Functional Components: These components handle specific tasks or logic, such as data fetching, state management, or API calls.
- Layout Components: These components define the structure and layout of a page, such as headers, footers, and sidebars.
- Composite Components: These are combinations of multiple components that work together to provide a more complex functionality.
Importance of Components in Software Development
Components play a crucial role in modern software development for several reasons:
- Reusability: Components can be reused across different parts of an application, reducing code duplication and saving development time.
- Maintainability: By encapsulating functionality within components, developers can easily update or fix issues without affecting the entire application.
- Scalability: Components make it easier to scale applications by allowing developers to add new features or modify existing ones without disrupting the overall structure.
- Collaboration: Components enable teams to work more efficiently by dividing the development process into smaller, manageable tasks.
2. Planning Your Component
Identifying the Need for a New Component
Before creating a new component, it’s essential to determine whether it’s necessary. Ask yourself the following questions:
- Is the functionality or UI element not already available in your project?
- Will the component be reused in multiple places?
- Does the component encapsulate a specific task or feature that can be isolated from the rest of the application?
If the answer to these questions is yes, then creating a new component is justified.
Defining the Component’s Purpose
Clearly define the purpose of your component. What problem does it solve? What functionality does it provide? Having a clear understanding of the component’s purpose will guide your design and implementation decisions.
Gathering Requirements
Once you’ve identified the need for a new component, gather all the necessary requirements. This includes:
- Functional Requirements: What should the component do? What inputs does it need, and what outputs should it produce?
- Non-Functional Requirements: What are the performance, accessibility, and usability expectations for the component?
- Design Requirements: What should the component look like? Are there any specific design guidelines or branding requirements?
Sketching the Component’s Design
Before diving into code, it’s helpful to sketch out the component’s design. This can be done using wireframing tools or even on paper. Sketching helps you visualize the component’s layout, structure, and interactions, making it easier to translate into code later.
3. Designing the Component
Choosing the Right Technology Stack
The technology stack you choose for your component will depend on the overall architecture of your application. For web development, common choices include:
- HTML/CSS/JavaScript: For basic UI components.
- React/Vue/Angular: For more complex, interactive components.
- Sass/Less: For styling components with pre-processors.
- TypeScript: For adding type safety to your JavaScript code.
Consider the pros and cons of each technology and choose the one that best fits your project’s needs.
Designing the Component’s Architecture
The architecture of your component refers to how it’s structured internally. A well-designed component should be:
- Modular: Break down the component into smaller, reusable parts if possible.
- Decoupled: Ensure that the component is independent of other parts of the application.
- Scalable: Design the component in a way that allows for future enhancements or modifications.
Creating a Mockup or Prototype
Once you’ve chosen your technology stack and designed the component’s architecture, create a mockup or prototype. This can be a static HTML/CSS mockup or an interactive prototype using a framework like React or Vue. The goal is to get a working version of the component that you can test and iterate on.
Considering User Experience (UX)
User experience is a critical aspect of component design. Consider the following UX principles when designing your component:
- Usability: Ensure that the component is easy to use and understand.
- Accessibility: Make sure the component is accessible to all users, including those with disabilities.
- Responsiveness: Design the component to work well on different screen sizes and devices.
- Performance: Optimize the component to load quickly and respond smoothly to user interactions.
4. Implementing the Component
Setting Up the Development Environment
Before writing any code, set up your development environment. This includes:
Setting Up Version Control: Use Git or another version control system to track changes to your code.
Installing Dependencies: Install any necessary libraries or frameworks.
Configuring Build Tools: Set up tools like Webpack, Babel, or Vite for bundling and transpiling your code.
Writing the Component’s Code
With your environment set up, you can start writing the code for your component. Follow these steps:
- Create the Component File: Start by creating a new file for your component. Depending on your framework, this could be a
.jsx
,.vue
, or.tsx
file. - Define the Component’s Structure: Write the HTML or JSX code that defines the structure of your component.
- Add Styles: Use CSS, Sass, or a CSS-in-JS solution to style your component.
- Implement Functionality: Add the necessary JavaScript or TypeScript code to handle the component’s logic and interactions.
- Handle Props and State: If your component needs to accept input or manage internal state, implement the necessary props and state management.
Testing the Component
Testing is a crucial step in the development process. Ensure that your component works as expected by writing unit tests, integration tests, and end-to-end tests. Use testing libraries like Jest, Enzyme, or Cypress to automate your tests and catch any issues early.
Integrating the Component into Your Application
Once your component is tested and working, integrate it into your application. This involves:
- Importing the Component: Import the component into the relevant part of your application.
- Passing Props: If your component accepts props, pass the necessary data from the parent component.
- Styling Integration: Ensure that the component’s styles integrate seamlessly with the rest of your application.
- Testing in Context: Test the component within the context of your application to ensure it works as expected.
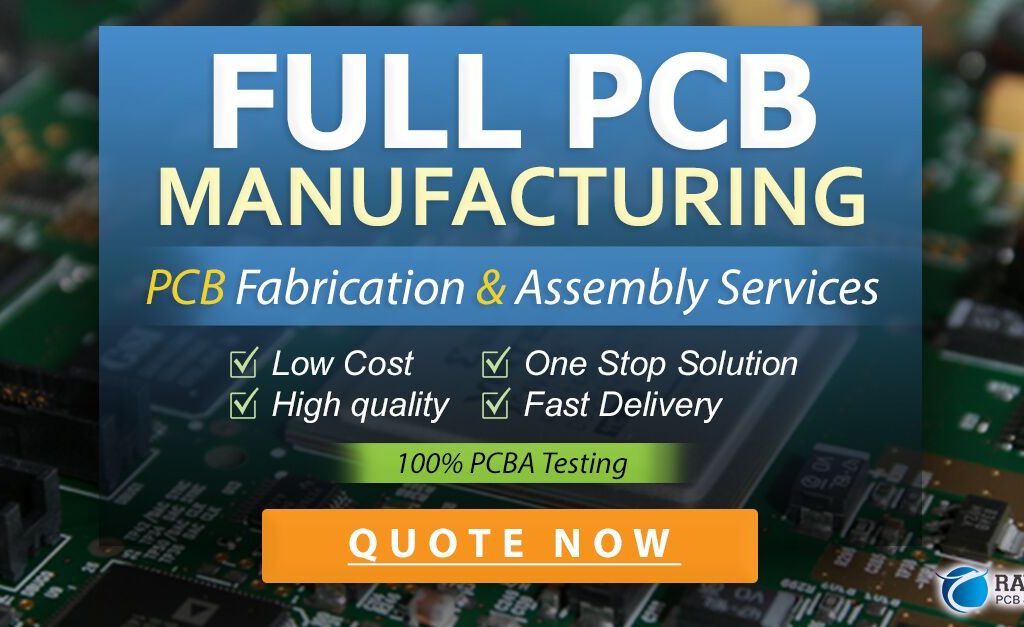
5. Best Practices for Component Development
Writing Clean and Maintainable Code
Clean code is essential for long-term maintainability. Follow these best practices:
- Use Descriptive Names: Choose meaningful names for your variables, functions, and components.
- Follow Coding Standards: Adhere to the coding standards and conventions of your team or framework.
- Keep Functions Small: Break down complex logic into smaller, reusable functions.
- Avoid Hardcoding: Use constants or configuration files for values that may change in the future.
Ensuring Reusability
Reusability is one of the main benefits of components. To ensure your component is reusable:
Document Usage: Clearly document how to use the component, including examples and prop descriptions.
Make it Generic: Avoid hardcoding specific data or logic that limits the component’s use cases.
Use Props: Allow the component to accept props that customize its behavior or appearance.
Provide Defaults: Provide default values for props to make the component easy to use out of the box.
Optimizing Performance
Performance is critical for a good user experience. Optimize your component by:
- Minimizing Re-renders: Use techniques like memoization or shouldComponentUpdate to prevent unnecessary re-renders.
- Lazy Loading: Load the component only when it’s needed, especially for large or complex components.
- Optimizing Assets: Compress images and other assets used in the component to reduce load times.
- Using Efficient Algorithms: Choose algorithms and data structures that are efficient and scalable.
Documenting Your Component
Documentation is essential for both your future self and other developers who may use your component. Include:
- Component Description: A brief overview of what the component does.
- Props and State: A list of all props and state variables, along with their types and descriptions.
- Usage Examples: Code examples showing how to use the component in different scenarios.
- API Reference: If your component exposes any methods or events, document them clearly.
6. Common Pitfalls and How to Avoid Them
Overcomplicating the Component
One common mistake is overcomplicating the component by adding unnecessary features or logic. To avoid this:
Iterate: Start with a minimal version of the component and add features as needed.
Keep it Simple: Focus on the core functionality and avoid adding features that aren’t essential.
YAGNI Principle: Follow the “You Aren’t Gonna Need It” principle and only implement what’s necessary.
Ignoring Cross-Browser Compatibility
Different browsers may render your component differently, leading to inconsistencies. To ensure cross-browser compatibility:
- Test on Multiple Browsers: Test your component on all major browsers, including Chrome, Firefox, Safari, and Edge.
- Use Polyfills: If you’re using modern JavaScript features, include polyfills to support older browsers.
- Check CSS Compatibility: Ensure that your CSS works across different browsers and use vendor prefixes if necessary.
Neglecting Accessibility
Accessibility is often overlooked but is crucial for making your component usable by everyone. To improve accessibility:
- Use Semantic HTML: Use appropriate HTML elements (e.g.,
<button>
,<input>
) to ensure screen readers can interpret your component correctly. - Add ARIA Attributes: Use ARIA (Accessible Rich Internet Applications) attributes to provide additional context for screen readers.
- Keyboard Navigation: Ensure that your component can be navigated using a keyboard alone.
- Contrast and Color: Use sufficient color contrast and avoid relying solely on color to convey information.
Failing to Test Thoroughly
Inadequate testing can lead to bugs and issues in production. To ensure thorough testing:
Test Edge Cases: Consider edge cases and unexpected inputs to ensure your component handles them gracefully.
Write Unit Tests: Test individual functions and methods to ensure they work as expected.
Write Integration Tests: Test how the component interacts with other parts of the application.
Write End-to-End Tests: Simulate user interactions to ensure the component behaves correctly in real-world scenarios.
7. Conclusion
Creating a new component is a multi-step process that involves planning, designing, implementing, and testing. By following best practices and avoiding common pitfalls, you can create components that are reusable, maintainable, and efficient. Remember to keep the user experience in mind, optimize for performance, and document your component thoroughly. With these guidelines, you’ll be well-equipped to create high-quality components that enhance your application and streamline your development process.
Recap of Key Points
- Components are self-contained, reusable pieces of code that encapsulate specific functionality or UI elements.
- Planning involves identifying the need for a new component, defining its purpose, gathering requirements, and sketching its design.
- Designing includes choosing the right technology stack, designing the component’s architecture, creating a mockup, and considering UX principles.
- Implementing involves setting up the development environment, writing the component’s code, testing, and integrating it into your application.
- Best Practices include writing clean and maintainable code, ensuring reusability, optimizing performance, and documenting your component.
- Common Pitfalls include overcomplicating the component, ignoring cross-browser compatibility, neglecting accessibility, and failing to test thoroughly.
Final Thoughts on Creating a New Component
Creating a new component is both an art and a science. It requires a balance of technical skills, creativity, and attention to detail. By following the steps outlined in this guide, you can create components that not only meet the immediate needs of your application but also stand the test of time. Whether you’re a seasoned developer or just starting out, mastering the art of component creation will make you a more effective and efficient developer. Happy coding!