Introduction to Thermocouples and Temperature Measurement
Accurate temperature measurement is crucial in a wide range of applications, from industrial processes to scientific experiments. Thermocouples are popular temperature sensors due to their wide measurement range, durability, and relatively low cost. However, converting the thermocouple’s analog signal into a digital value that can be easily read and processed by a microcontroller or computer requires a specialized circuit called a Thermocouple Converter.
What is a Thermocouple?
A thermocouple is a temperature sensor that consists of two dissimilar metal wires joined together at one end, forming a junction. When the junction is exposed to a temperature different from the reference temperature at the other end of the wires, a voltage is generated. This voltage, known as the Seebeck voltage, is proportional to the temperature difference between the two ends.
Thermocouples are available in various types, each with a specific combination of metals and suitable for different temperature ranges and environments. The most common thermocouple types are:
Type | Positive Metal | Negative Metal | Temperature Range |
---|---|---|---|
J | Iron | Constantan | -210°C to 1200°C |
K | Chromel | Alumel | -270°C to 1372°C |
T | Copper | Constantan | -270°C to 400°C |
E | Chromel | Constantan | -270°C to 1000°C |
N | Nicrosil | Nisil | -270°C to 1300°C |
Challenges in Thermocouple Signal Conditioning
While thermocouples are simple and effective temperature sensors, their output signal is relatively small (in the millivolt range) and requires amplification and cold-junction compensation (CJC) to obtain an accurate temperature reading. Additionally, the thermocouple’s output is non-linear, meaning that the relationship between the Seebeck voltage and the temperature is not a straight line.
To address these challenges and simplify the process of reading temperatures from thermocouples, engineers use thermocouple converters like the MAX31856.
Understanding the MAX31856 Thermocouple Converter
The MAX31856 is a precision thermocouple-to-digital converter designed by Maxim Integrated. It combines an analog front-end, ADC, digital signal processing, and a SPI-compatible interface in a single chip, making it easy to integrate with microcontrollers and other digital systems.
Key Features of the MAX31856
- Supports multiple thermocouple types: J, K, N, R, S, T, E, and B
- Cold-junction compensation with internal temperature sensor
- High-resolution ADC (19 bits)
- Linearization and error correction
- SPI-compatible interface (up to 5 MHz)
- Wide operating voltage range (3.0V to 3.6V)
- Fault detection for open and shorted thermocouples
- Programmable alert thresholds
MAX31856 Block Diagram
The MAX31856 consists of several key components that work together to convert the thermocouple’s analog signal into a digital value:
[Insert block diagram image here]
- Analog Front-End (AFE): Amplifies and filters the thermocouple’s small analog signal.
- Analog-to-Digital Converter (ADC): Converts the amplified analog signal into a digital value.
- Digital Signal Processor (DSP): Performs linearization, cold-junction compensation, and error correction.
- SPI Interface: Allows communication between the MAX31856 and a microcontroller or other digital system.
Configuring the MAX31856
The MAX31856 is highly configurable, allowing users to select the thermocouple type, set the ADC resolution, and adjust various other settings through the SPI interface. The chip has several registers that can be read and written to configure its operation:
Register | Address | Description |
---|---|---|
CR0 | 0x00 | Configuration register 0 |
CR1 | 0x01 | Configuration register 1 |
MASK | 0x02 | Fault mask register |
CJHF | 0x03 | Cold-junction high fault threshold |
CJLF | 0x04 | Cold-junction low fault threshold |
LTHFTH | 0x05 | Linearized temperature high fault threshold (MSB) |
LTHFTL | 0x06 | Linearized temperature high fault threshold (LSB) |
LTLFTH | 0x07 | Linearized temperature low fault threshold (MSB) |
LTLFTL | 0x08 | Linearized temperature low fault threshold (LSB) |
CJTO | 0x09 | Cold-junction temperature offset |
CJTH | 0x0A | Cold-junction temperature register (MSB) |
CJTL | 0x0B | Cold-junction temperature register (LSB) |
LTCBH | 0x0C | Linearized TC temperature register (Byte 2) |
LTCBM | 0x0D | Linearized TC temperature register (Byte 1) |
LTCBL | 0x0E | Linearized TC temperature register (Byte 0) |
SR | 0x0F | Status register |
For example, to configure the MAX31856 for a K-type thermocouple with 19-bit resolution, you would write the appropriate values to the CR0 and CR1 registers:
CR0 = 0x03 // K-type thermocouple, 19-bit resolution
CR1 = 0x00 // No averaging, no fault detection
Interfacing the MAX31856 with a Microcontroller
To read temperature values from the MAX31856, you need to connect it to a microcontroller or other digital system using the SPI interface. The MAX31856 requires four connections:
- SCLK: Serial clock input
- CS: Chip select input (active low)
- SDI: Serial data input
- SDO: Serial data output
[Insert wiring diagram image here]
Reading Temperature Values
To read the cold-junction and thermocouple temperatures from the MAX31856, follow these steps:
- Configure the MAX31856 by writing to the appropriate registers (e.g., CR0 and CR1).
- Read the cold-junction temperature registers (CJTH and CJTL) and combine the values to obtain the cold-junction temperature in degrees Celsius.
- Read the linearized thermocouple temperature registers (LTCBH, LTCBM, and LTCBL) and combine the values to obtain the thermocouple temperature in degrees Celsius.
Here’s an example of how to read the temperature values using an Arduino:
#include <SPI.h>
const int CS_PIN = 10;
void setup() {
SPI.begin();
pinMode(CS_PIN, OUTPUT);
digitalWrite(CS_PIN, HIGH);
// Configure MAX31856 for K-type thermocouple, 19-bit resolution
writeRegister(0x00, 0x03);
writeRegister(0x01, 0x00);
}
void loop() {
// Read cold-junction temperature
int cjTemp = readCJTemperature();
// Read thermocouple temperature
int tcTemp = readTCTemperature();
// Print temperatures
Serial.print("Cold-Junction Temperature: ");
Serial.print(cjTemp);
Serial.print(" °C, Thermocouple Temperature: ");
Serial.print(tcTemp);
Serial.println(" °C");
delay(1000);
}
int readCJTemperature() {
int cjth = readRegister(0x0A);
int cjtl = readRegister(0x0B);
int cjt = ((cjth << 8) | cjtl) >> 2;
return cjt * 0.015625; // Convert to degrees Celsius
}
int readTCTemperature() {
int ltcbh = readRegister(0x0C);
int ltcbm = readRegister(0x0D);
int ltcbl = readRegister(0x0E);
int ltcb = ((ltcbh << 16) | (ltcbm << 8) | ltcbl) >> 5;
return ltcb * 0.0078125; // Convert to degrees Celsius
}
void writeRegister(byte reg, byte value) {
digitalWrite(CS_PIN, LOW);
SPI.transfer(reg & 0x7F);
SPI.transfer(value);
digitalWrite(CS_PIN, HIGH);
}
byte readRegister(byte reg) {
digitalWrite(CS_PIN, LOW);
SPI.transfer(reg | 0x80);
byte value = SPI.transfer(0x00);
digitalWrite(CS_PIN, HIGH);
return value;
}
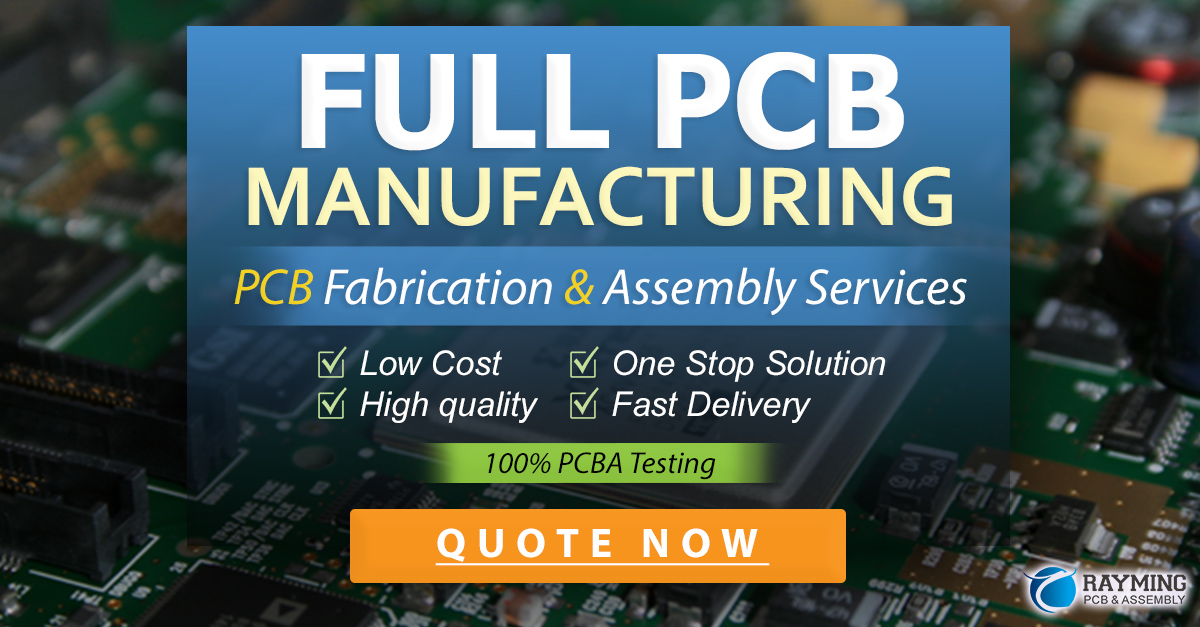
MAX31856 Fault Detection and Handling
The MAX31856 includes built-in fault detection for open and shorted thermocouples, as well as out-of-range temperatures. When a fault occurs, the corresponding bit is set in the status register (SR), and the FAULT pin is pulled low.
To enable fault detection, set the appropriate bits in the fault mask register (MASK) and configuration register 1 (CR1). For example, to enable open-circuit fault detection:
MASK = 0x80 // Enable open-circuit fault detection
CR1 |= 0x40 // Enable fault mode
To check for faults, read the status register (SR) and check the relevant bits:
Bit | Description |
---|---|
7 | Cold-junction high fault |
6 | Cold-junction low fault |
5 | Thermocouple temperature high fault |
4 | Thermocouple temperature low fault |
1 | Overvoltage or undervoltage fault |
0 | Open-circuit fault |
If a fault is detected, take appropriate action, such as notifying the user or implementing a failsafe mechanism.
Conclusion
The MAX31856 is a powerful and versatile thermocouple converter that simplifies the process of reading temperatures from thermocouples. By integrating cold-junction compensation, linearization, and error correction, the MAX31856 provides accurate temperature measurements with minimal external components.
When designing a temperature monitoring system using the MAX31856, consider the following:
- Choose the appropriate thermocouple type for your application based on the temperature range and environment.
- Configure the MAX31856 settings, such as ADC resolution and fault detection, to meet your system requirements.
- Implement fault handling to ensure the reliability and safety of your temperature monitoring system.
By understanding the capabilities and configuration of the MAX31856, you can create robust and accurate temperature monitoring solutions for a wide range of applications.
FAQ
1. What is the difference between the MAX31856 and other thermocouple converters?
The MAX31856 is a highly integrated thermocouple converter that combines an analog front-end, ADC, digital signal processing, and SPI interface in a single chip. This integration simplifies the design process and reduces the number of external components required. The MAX31856 also supports a wide range of thermocouple types and includes advanced features like fault detection and programmable alert thresholds.
2. Can the MAX31856 be used with thermocouples other than the supported types (J, K, N, R, S, T, E, and B)?
While the MAX31856 is designed to work with the supported thermocouple types, it may be possible to use it with other types by implementing custom linearization and error correction algorithms. However, this requires significant effort and may not provide the same level of accuracy as using a supported thermocouple type.
3. How does the MAX31856 handle cold-junction compensation?
The MAX31856 includes an internal temperature sensor that measures the temperature at the cold junction (the point where the thermocouple wires connect to the MAX31856). This temperature is used to compensate for the voltage generated at the cold junction, allowing the MAX31856 to provide an accurate measurement of the temperature at the thermocouple tip.
4. What is the maximum sampling rate of the MAX31856?
The MAX31856’s maximum sampling rate depends on the selected ADC resolution and the SPI clock speed. With a 5 MHz SPI clock and 19-bit resolution, the maximum sampling rate is approximately 15 samples per second. Lower resolutions and higher SPI clock speeds can increase the sampling rate, but may reduce the accuracy of the temperature measurements.
5. How do I set the alert thresholds for the MAX31856?
The MAX31856 allows you to set high and low temperature thresholds for both the cold-junction and thermocouple temperatures. When the measured temperature exceeds these thresholds, the MAX31856 sets the corresponding bit in the status register and pulls the ALERT pin low. To set the thresholds, write the desired values to the CJHF, CJLF, LTHFTH, LTHFTL, LTLFTH, and LTLFTL registers.