Introduction to the DS1307 Real-Time Clock Module
The DS1307 is a popular real-time clock (RTC) module that provides accurate timekeeping for Arduino projects. This low-power chip can keep track of seconds, minutes, hours, days, months, and years, with automatic leap year compensation. The DS1307 communicates with the Arduino using the I2C protocol, making it easy to integrate into your projects.
Key Features of the DS1307
- Accurate timekeeping with seconds, minutes, hours, days, months, and years
- Automatic leap year compensation
- Low power consumption
- I2C interface for easy communication with Arduino
- 56-byte non-volatile RAM for data storage
- Optional square wave output
How the DS1307 Works
The DS1307 uses a crystal oscillator to maintain accurate time. The chip contains a battery-backed RAM that stores the current time and date, even when the main power is off. When the DS1307 is powered on, it reads the time and date from the RAM and continues counting from that point.
The DS1307 communicates with the Arduino using the I2C protocol. I2C (Inter-Integrated Circuit) is a synchronous, multi-master, multi-slave, packet switched, single-ended, serial communication bus. It uses two wires for data transfer: SCL (Serial Clock) and SDA (Serial Data). The Arduino acts as the master device, initiating communication with the DS1307 and requesting time and date information.
DS1307 Pinout
Pin | Name | Description |
---|---|---|
1 | X1 | 32.768 kHz crystal connection |
2 | X2 | 32.768 kHz crystal connection |
3 | VBAT | +3V battery input for timekeeping when main power is off |
4 | GND | Ground |
5 | SDA | Serial Data (I2C) |
6 | SCL | Serial Clock (I2C) |
7 | SQW/OUT | Square Wave/Output Driver |
8 | VCC | +5V power supply |
Interfacing the DS1307 with Arduino
To interface the DS1307 with an Arduino, you’ll need to make the following connections:
DS1307 Pin | Arduino Pin |
---|---|
VCC | 5V |
GND | GND |
SDA | A4 (or SDA) |
SCL | A5 (or SCL) |
Step 1: Install the RTClib Library
To simplify working with the DS1307, we’ll use the RTClib library by Adafruit. You can install this library using the Arduino IDE’s Library Manager:
- Open the Arduino IDE
- Go to Sketch > Include Library > Manage Libraries
- Search for “RTClib” in the search bar
- Select the “RTClib by Adafruit” library and click “Install”
Step 2: Set the Current Time and Date
Before you can use the DS1307 to keep track of time, you need to set the current time and date. Here’s an example sketch that demonstrates how to do this:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
if (!rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
// Set the current time and date (uncomment the line below and modify as needed)
// rtc.adjust(DateTime(2023, 4, 14, 10, 30, 0));
}
void loop() {
DateTime now = rtc.now();
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(' ');
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.print(now.second(), DEC);
Serial.println();
delay(1000);
}
To set the current time and date, uncomment the line rtc.adjust(DateTime(2023, 4, 14, 10, 30, 0));
in the setup()
function and modify the values to match the current date and time. Upload the sketch to your Arduino, and the DS1307 will be set with the specified time and date.
Step 3: Reading Time and Date from the DS1307
Once the DS1307 is set with the current time and date, you can read the time and date information in your Arduino sketches. Here’s an example sketch that demonstrates how to read and display the time and date:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
if (!rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
}
void loop() {
DateTime now = rtc.now();
Serial.print("Date: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.println(now.day(), DEC);
Serial.print("Time: ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
delay(1000);
}
This sketch reads the current time and date from the DS1307 using the rtc.now()
function and displays the information in a user-friendly format on the Serial Monitor.
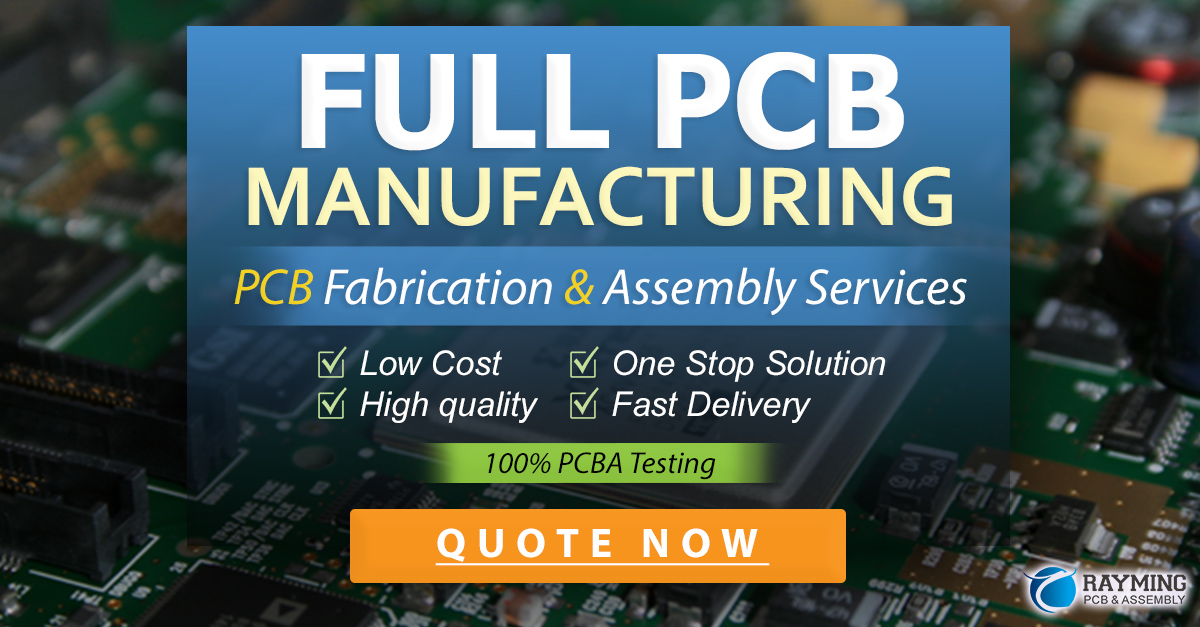
Troubleshooting Common Issues
If you encounter problems while working with the DS1307 and Arduino, here are a few common issues and their solutions:
DS1307 Not Detected
If the Arduino sketch reports that it couldn’t find the RTC, check the following:
- Ensure that the DS1307 is properly connected to the Arduino, with the correct pin assignments.
- Verify that the DS1307 is functioning correctly by testing it with a multimeter.
- Check that the RTClib library is correctly installed in your Arduino IDE.
Incorrect Time or Date
If the time or date displayed by the DS1307 is incorrect, try the following:
- Ensure that you have set the correct time and date using the
rtc.adjust()
function. - Check that the DS1307’s battery is functioning correctly and providing backup power when the main power is off.
- Verify that the crystal oscillator is properly connected and functioning.
Project Ideas Using the DS1307 and Arduino
Here are a few project ideas that demonstrate the capabilities of the DS1307 and Arduino:
- Digital Clock: Create a digital clock that displays the current time and date on an LCD or OLED display.
- Time-Based Controller: Use the DS1307 to control devices based on time, such as turning lights on and off at specific times or triggering events at predefined intervals.
- Data Logger: Combine the DS1307 with sensors and an SD card module to create a data logger that records sensor readings along with a timestamp.
- Attendance System: Build an attendance system that logs the time and date when employees or students check in using an RFID reader or keypad.
- Programmable Timer: Create a programmable timer that allows users to set custom time intervals for various tasks or reminders.
Conclusion
The DS1307 is a versatile and easy-to-use real-time clock module that can greatly enhance your Arduino projects. By providing accurate timekeeping and the ability to store data even when the main power is off, the DS1307 opens up a wide range of possibilities for time-based applications. With the RTClib library and the interfacing techniques covered in this article, you’ll be well-equipped to incorporate the DS1307 into your own projects.
Frequently Asked Questions (FAQ)
- Q: Can the DS1307 keep track of time without a backup battery?
A: No, the DS1307 requires a backup battery (typically a 3V coin cell) to maintain timekeeping when the main power is off. Without a battery, the DS1307 will lose its time and date settings when power is removed. - Q: How accurate is the DS1307’s timekeeping?
A: The DS1307’s accuracy depends on the quality of the crystal oscillator and environmental factors such as temperature. Typically, the DS1307 has an accuracy of ±2ppm at 25°C, which translates to about ±1 minute per year. - Q: Can I use the DS1307 with other microcontrollers besides the Arduino?
A: Yes, the DS1307 uses the standard I2C protocol, which is supported by many microcontrollers. You can use the DS1307 with any microcontroller that has I2C capabilities, such as the Raspberry Pi, ESP32, or PIC microcontrollers. - Q: How do I set the initial time and date on the DS1307?
A: To set the initial time and date, you need to use thertc.adjust()
function provided by the RTClib library. Pass aDateTime
object with the desired time and date to this function, and the DS1307 will be set accordingly. - Q: Can I use multiple DS1307 modules with a single Arduino?
A: Yes, you can use multiple DS1307 modules with a single Arduino by assigning each module a unique I2C address. The DS1307 has a fixed I2C address of 0x68, but you can modify the address by connecting the SQW/OUT pin to VCC, which changes the address to 0x69.